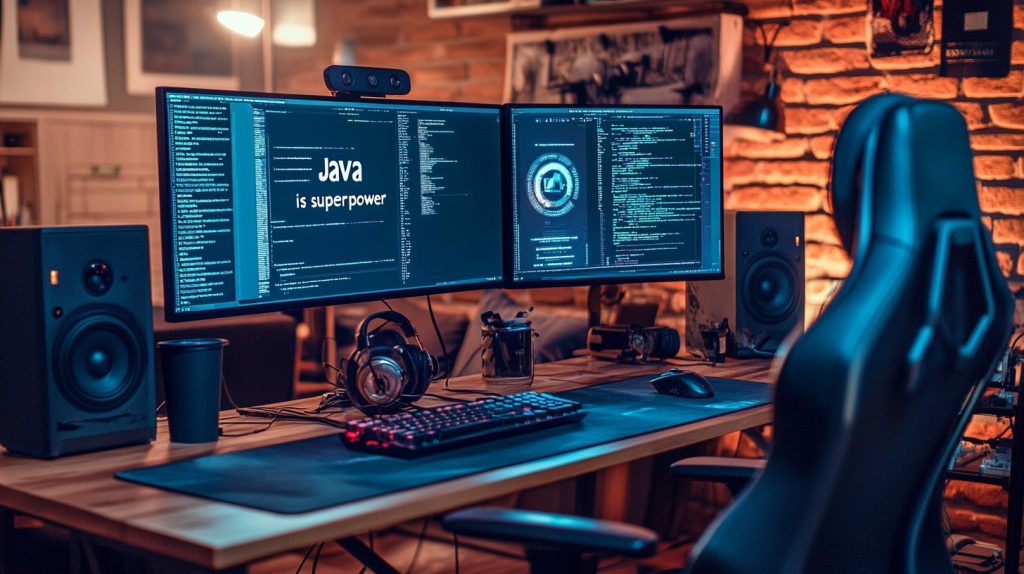
Java Roadmap
Mastering Java requires a step-by-step approach, moving from the basics to advanced topics. Here’s a streamlined roadmap to guide your journey:
1. Setup and Tools
- Linux: Learn basic commands.
- Git: Master version control for collaboration.
- IDEs: Familiarize yourself with:
- IntelliJ IDEA, Eclipse, or VSCode.
2. Core Java Concepts
- OOP: Understand classes, objects, inheritance, and polymorphism.
- Arrays & Strings: Work with data structures and string manipulation.
- Loops: Control flow with
for
,while
, anddo-while
. - Interfaces & Packages: Organize and structure code.
3. File I/O and Collections
- File Handling: Learn file operations using I/O Streams.
- Collections Framework: Work with Lists, Maps, Stacks, and Queues.
- Optionals: Avoid null pointer exceptions with
Optional
.
4. Advanced Java Concepts
- Dependency Injection: Understand DI patterns.
- Design Patterns: Learn common patterns like Singleton and Factory.
- JVM Internals: Learn memory management and garbage collection.
- Multi-Threading: Handle concurrency and threads.
- Generics & Exception Handling: Write type-safe code and handle errors gracefully.
- Streams: Work with functional programming using Streams.
5. Testing and Debugging
- Unit & Integration Testing: Use JUnit/TestNG for testing.
- Debugging: Learn debugging techniques.
- Mocking: Use libraries like Mockito for test isolation.
6. Databases
- Database Design: Learn to design schemas and write efficient queries.
- SQL & NoSQL: Work with relational (JDBC) and non-relational databases.
- Schema Migration Tools: Use Flyway or Liquibase for migrations.
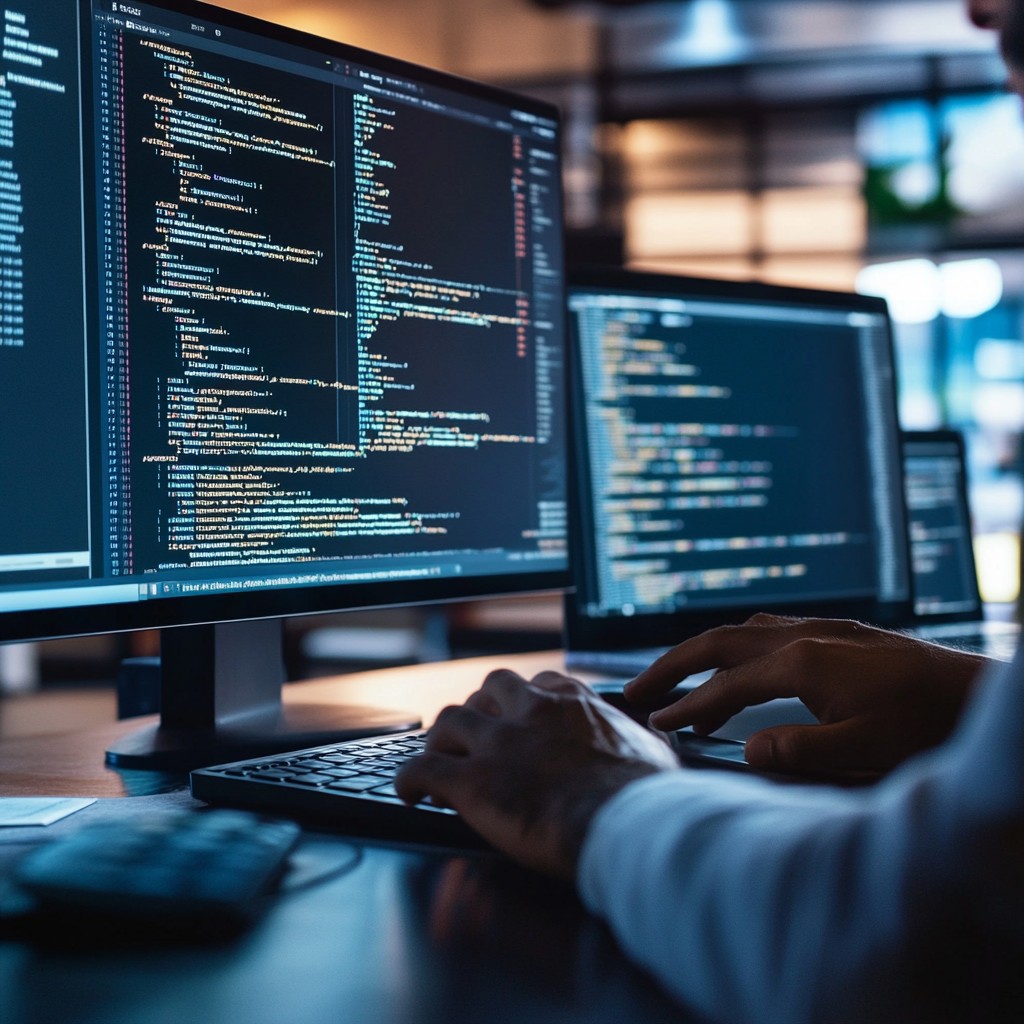
7. Clean Code Practices
- SOLID Principles: Write maintainable and scalable code.
- Immutability: Ensure thread-safe and predictable objects.
- Logging: Implement effective logging for debugging.
8. Build Tools
- Learn to use Maven, Gradle, or Bazel for project builds.
9. HTTP and APIs
- HTTP Protocol & REST API: Design scalable APIs.
- GraphQL: Explore efficient querying with GraphQL.
10. Frameworks
- Spring Boot: Build production-ready applications.
- Play & Quarkus: Learn lightweight, cloud-native frameworks.
Let us develop your Java application!
Let us know at hello@cyberwhale.tech